diff --git a/src/App.tsx b/src/App.tsx
index 9945376..6b448a1 100644
--- a/src/App.tsx
+++ b/src/App.tsx
@@ -6,8 +6,10 @@ function App() {
return (
-
-
test
+
+
test
+
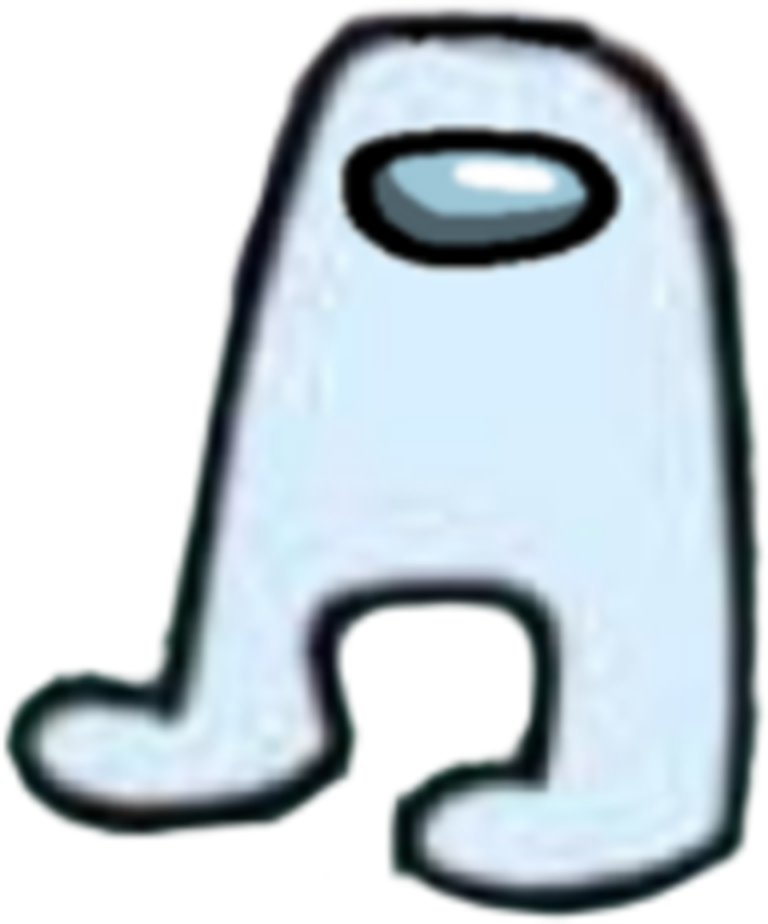
+
test 2
);
diff --git a/src/Navbar.tsx b/src/Navbar.tsx
index bfa9fc1..0a5993e 100644
--- a/src/Navbar.tsx
+++ b/src/Navbar.tsx
@@ -1,6 +1,8 @@
import { FunctionComponent } from 'react';
import { useState, useEffect } from 'react';
import './navbar.css';
+import NavbarLink from './NavbarLink';
+import NavbarLinkDivider from './NavbarLinkDivider';
import NavbarText from './NavbarText';
const Navbar: FunctionComponent = () => {
@@ -20,6 +22,11 @@ const Navbar: FunctionComponent = () => {
return (
)
}
diff --git a/src/NavbarLink.tsx b/src/NavbarLink.tsx
new file mode 100644
index 0000000..79a0022
--- /dev/null
+++ b/src/NavbarLink.tsx
@@ -0,0 +1,9 @@
+import { FunctionComponent } from "react";
+
+const NavbarLink: FunctionComponent<{ text: string, link: string }> = (props) => {
+ return (
+ window.location.href = props.link}>{props.text}
+ );
+}
+
+export default NavbarLink;
diff --git a/src/NavbarLinkDivider.tsx b/src/NavbarLinkDivider.tsx
new file mode 100644
index 0000000..6a94eab
--- /dev/null
+++ b/src/NavbarLinkDivider.tsx
@@ -0,0 +1,16 @@
+import { FunctionComponent } from "react";
+
+const NavbarLinkDivider: FunctionComponent = (props) => {
+ return (
+ |
+ );
+}
+
+export default NavbarLinkDivider;
diff --git a/src/NavbarText.tsx b/src/NavbarText.tsx
index 64b705a..37f3b23 100644
--- a/src/NavbarText.tsx
+++ b/src/NavbarText.tsx
@@ -7,8 +7,14 @@ const Texts: string[] = [
'jan://',
'./jan',
'/usr/bin/jan',
+ '/usr/lib/libjan-0.so.14',
+ 'https://rvlt.gg/jan',
];
+const HrefOverrides: { [key: number]: string } = {
+ 5: 'https://rvlt.gg/jan',
+}
+
const randomText = (excludeNum?: number): number => {
if (excludeNum === undefined || Texts.length === 1) return Math.floor(Math.random() * Texts.length);
else {
@@ -30,11 +36,11 @@ const NavbarText: FunctionComponent = () => {
if (text.length < Texts[selectedText].length) {
setText(text + Texts[selectedText].charAt(text.length));
}
-
+
if (timer % 5 === 0) {
setUnderscore(!underscore);
}
-
+
if (timer >= 80) {
if (text.length > 0) {
setText(text.substring(0, text.length - 1));
@@ -57,7 +63,7 @@ const NavbarText: FunctionComponent = () => {
return (
- window.location.href = '/'}>
+ window.location.href = HrefOverrides[selectedText] || '/'}>
{'> '}{text}{underscore ? '_' : ''}
diff --git a/src/index.css b/src/index.css
index 482d7a4..9765afc 100644
--- a/src/index.css
+++ b/src/index.css
@@ -3,6 +3,10 @@
--secondary-bg: #232323;
}
+html {
+ scroll-behavior: smooth;
+}
+
body {
margin: 0;
font-family: 'Roboto', sans-serif;
diff --git a/src/navbar.css b/src/navbar.css
index beaaad4..02ea182 100644
--- a/src/navbar.css
+++ b/src/navbar.css
@@ -3,21 +3,26 @@
height: 64px;
top: 0;
position: fixed;
- background-color: var(--secondary-bg);
border-radius: 0 0 15px 15px;
text-shadow: 0 0 3px rgba(255, 255, 255, 0.85), 0 0 6px rgba(255, 255, 255, 0.45), 0 0 9px rgba(255, 255, 255, 0.3);
- user-select: none;
- cursor: pointer;
-
- transition: top 0.2s, text-shadow 0.3s;
-}
-
-.navbar:hover {
- text-shadow: 0 0 5px rgba(255, 255, 255, 0.85), 0 0 10px rgba(255, 255, 255, 0.45), 0 0 15px rgba(255, 255, 255, 0.3);
+ transition: height 0.2s;
}
.navbar-collapsed {
- top: -64px;
+ height: 32px;
+}
+
+.navbar-collapsed .navbar-text, .navbar-collapsed .navbar-link-text {
+ font-size: 16px;
+ top: 6px;
+}
+
+.navbar-collapsed .navbar-link-text {
+ right: 4px;
+}
+
+.navbar-text:hover, .navbar-link-text:hover {
+ text-shadow: 0 0 5px rgba(255, 255, 255, 0.85), 0 0 10px rgba(255, 255, 255, 0.45), 0 0 15px rgba(255, 255, 255, 0.3);
}
.navbar-text {
@@ -26,4 +31,34 @@
position: relative;
top: 8px;
left: 12px;
+ user-select: none;
+ cursor: pointer;
+ float: left;
+ transition: text-shadow 0.3s, font-size 0.2s;
+}
+
+.navbar-link-text {
+ position: relative;
+ top: 16px;
+ right: 16px;
+ font-size: 24px;
+ font-family: 'Quicksand', sans-serif;
+ text-align: center;
+ width: 100%;
+ padding-left: 6px;
+ padding-top: 8px;
+ user-select: none;
+ cursor: pointer;
+ transition: text-shadow 0.3s, font-size .2s, opacity .5s, top .2s, right .2s;
+}
+
+.navbar-link-container {
+ float: right;
+ top: 0;
+ right: 0;
+ transition: opacity .5s;
+}
+
+.navbar-collapsed .navbar-link-container {
+ opacity: .7;
}